Описание
Учитывая запятыми строку, если много цифры или строку с одним многозначным числом, вы можете использовать это регулярное выражение для замены ведущей запятой, а числа, равномерно делящиеся на 3
или 5
с нулевым значением.
(?:,|^)((?:[0369]|[258][0369]*[147]|[147](?:[0369]|[147][0369]*[258])*[258]|[258][0369]*[258](?:[0369]|[147][0369]*[258])*[258]|[147](?:[0369]|[147][0369]*[258])*[147][0369]*[147]|[258][0369]*[258](?:[0369]|[147][0369]*[258])*[147][0369]*[147])*|[0-9]*[50])(?=,|\Z)
более подробное объяснение этого выражения можно найти здесь: смотри также link
Заменить:
ничего
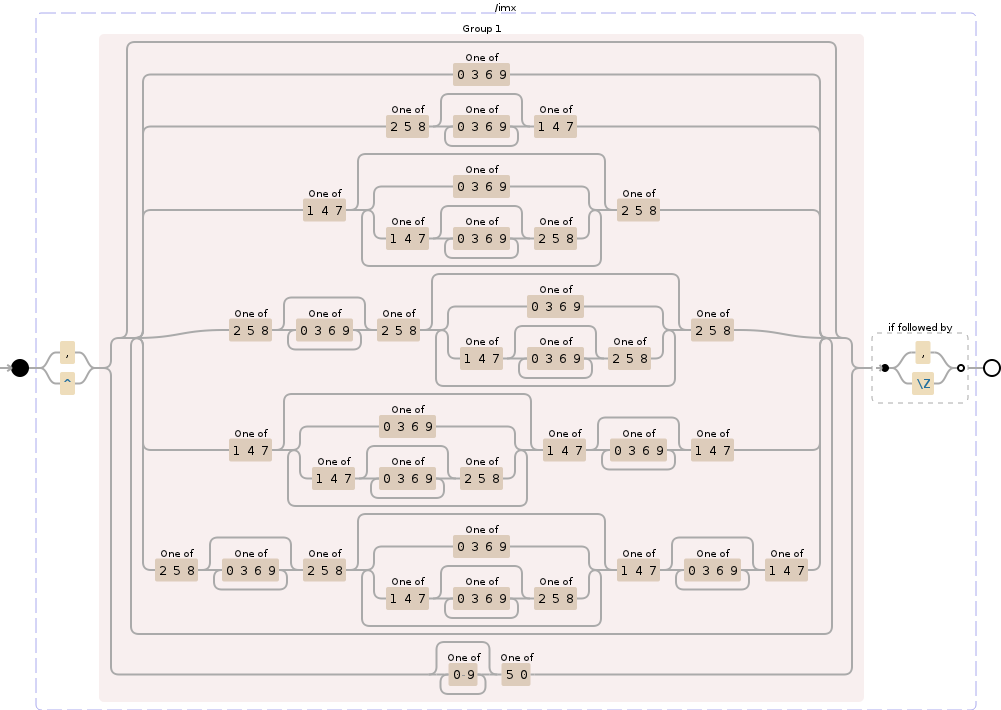
Пример
Демо
https://regex101.com/r/iG9lP4/1
Пример строки
1,2,3,5,9,15,16,21,23,25
После замены
1,2,16,23
Объяснение
NODE EXPLANATION
----------------------------------------------------------------------
(?: group, but do not capture:
----------------------------------------------------------------------
, ','
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
^ the beginning of a "line"
----------------------------------------------------------------------
) end of grouping
----------------------------------------------------------------------
( group and capture to \1:
----------------------------------------------------------------------
(?: group, but do not capture (0 or more
times (matching the most amount
possible)):
----------------------------------------------------------------------
[0369] any character of: '0', '3', '6', '9'
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
[0369]* any character of: '0', '3', '6', '9'
(0 or more times (matching the most
amount possible))
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
(?: group, but do not capture (0 or more
times (matching the most amount
possible)):
----------------------------------------------------------------------
[0369] any character of: '0', '3', '6', '9'
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
[0369]* any character of: '0', '3', '6', '9'
(0 or more times (matching the most
amount possible))
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
)* end of grouping
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
[0369]* any character of: '0', '3', '6', '9'
(0 or more times (matching the most
amount possible))
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
(?: group, but do not capture (0 or more
times (matching the most amount
possible)):
----------------------------------------------------------------------
[0369] any character of: '0', '3', '6', '9'
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
[0369]* any character of: '0', '3', '6', '9'
(0 or more times (matching the most
amount possible))
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
)* end of grouping
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
(?: group, but do not capture (0 or more
times (matching the most amount
possible)):
----------------------------------------------------------------------
[0369] any character of: '0', '3', '6', '9'
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
[0369]* any character of: '0', '3', '6', '9'
(0 or more times (matching the most
amount possible))
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
)* end of grouping
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
[0369]* any character of: '0', '3', '6', '9'
(0 or more times (matching the most
amount possible))
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
[0369]* any character of: '0', '3', '6', '9'
(0 or more times (matching the most
amount possible))
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
(?: group, but do not capture (0 or more
times (matching the most amount
possible)):
----------------------------------------------------------------------
[0369] any character of: '0', '3', '6', '9'
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
[0369]* any character of: '0', '3', '6', '9'
(0 or more times (matching the most
amount possible))
----------------------------------------------------------------------
[258] any character of: '2', '5', '8'
----------------------------------------------------------------------
)* end of grouping
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
[0369]* any character of: '0', '3', '6', '9'
(0 or more times (matching the most
amount possible))
----------------------------------------------------------------------
[147] any character of: '1', '4', '7'
----------------------------------------------------------------------
)* end of grouping
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
[0-9]* any character of: '0' to '9' (0 or more
times (matching the most amount
possible))
----------------------------------------------------------------------
[50] any character of: '5', '0'
----------------------------------------------------------------------
) end of \1
----------------------------------------------------------------------
(?= look ahead to see if there is:
----------------------------------------------------------------------
, ','
----------------------------------------------------------------------
| OR
----------------------------------------------------------------------
\Z before an optional \n, and the end of
the string
----------------------------------------------------------------------
) end of look-ahead
----------------------------------------------------------------------
Вам нужно ** перебрать ** по массиву. Вы можете сделать это, используя цикл '' for' (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/for). С какими особенностями у вас возникают проблемы? Мы будем рады помочь вам исправить ваш код, но мы не пишем код для вас. –
Возможный дубликат [Удалить конкретный элемент из массива в JavaScript?] (Http://stackoverflow.com/questions/5767325/remove-a-particular-element-from-an-array-in-javascript) – AlwaysNull
Вы можете также сделайте это с помощью [.filter] (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter) –